Introduction
Sorting algorithms form the backbone of computer science, playing a crucial role in organizing data efficiently and effectively. Among the myriad of sorting techniques available, Quicksort and Mergesort stand out due to their distinct advantages and widespread use. Quicksort is renowned for its speed and efficiency, particularly with large datasets, due to its divide-and-conquer approach and in-place sorting capabilities. On the other hand, Mergesort boasts stable sorting with consistent O(n log n) time complexity, making it a robust choice for handling data with predictable performance needs. However, each of these algorithms has its own set of drawbacks. Quicksort, despite its average-case efficiency, can degrade to O(n^2) time complexity in the worst case, while Mergesort, although consistent, requires additional space for its merge operations.
The quest for an optimal sorting solution has led to the development of hybrid algorithms that leverage the strengths of multiple techniques while mitigating their weaknesses. One such innovative approach is the hybrid sorting algorithm that combines Quicksort and Mergesort. This algorithm aims to harness the rapid partitioning capabilities of Quicksort for larger datasets while switching to the stable and predictable performance of Mergesort for smaller partitions. By integrating these two algorithms, the hybrid approach seeks to deliver superior performance, particularly in scenarios where the dataset size and nature vary significantly.
In this article, we will delve into the intricacies of implementing a hybrid sorting algorithm using pseudocode. We will explore how to seamlessly blend Quicksort and Mergesort, ensuring that the algorithm efficiently handles various dataset sizes and structures. The goal is to provide a detailed blueprint that can be easily translated into actual code, offering a practical solution for developers and computer scientists looking to optimize their sorting operations. By understanding the principles and mechanics behind this hybrid approach, readers will gain valuable insights into advanced sorting techniques and their applications in real-world scenarios.
We will begin by revisiting the fundamental concepts of Quicksort and Mergesort, highlighting their strengths and limitations. Following this, we will introduce the hybrid sorting algorithm, explaining the rationale behind combining these two methods. The pseudocode will be presented step-by-step, with thorough explanations to ensure clarity and comprehension. Additionally, we will discuss the importance of choosing an appropriate threshold for switching between Quicksort and Mergesort, a critical factor in optimizing the hybrid algorithm’s performance.
As we navigate through the implementation details, we will also touch upon the potential challenges and considerations that may arise. These include managing recursive calls, handling edge cases, and ensuring that the algorithm remains efficient in terms of both time and space complexity. Furthermore, we will compare the performance of the hybrid algorithm against standalone Quicksort and Mergesort implementations, providing empirical evidence of its advantages.
By the end of this article, readers will not only have a comprehensive understanding of the hybrid sorting algorithm but also the skills to implement it in their own projects. The combination of theoretical knowledge and practical application will empower developers to tackle complex sorting tasks with confidence and precision.
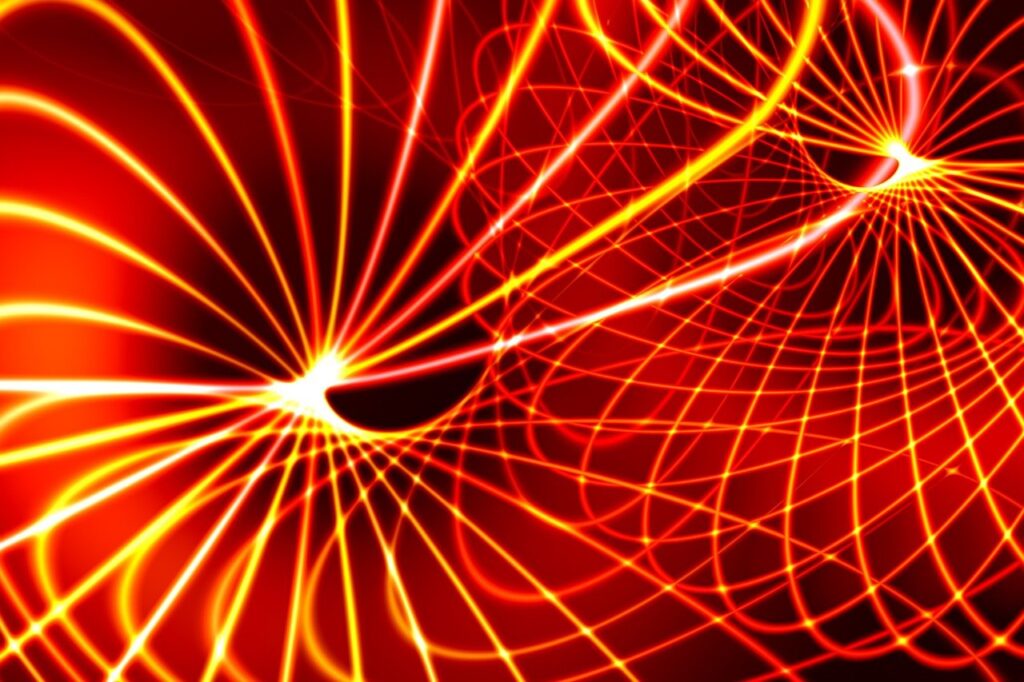
Pseudo code for a hybrid sorting algorithm
Below is the pseudocode for a hybrid sorting algorithm that combines Quicksort and Mergesort. The idea is to use Quicksort for larger subarrays and switch to Mergesort for smaller ones, leveraging the advantages of both algorithms.
Certainly! Here’s the detailed explanation with correct line numbers:
Pseudocode with Line Numbers and Detailed Explanation
1. // Function to implement the hybrid sorting algorithm
2. function hybridSort(arr, low, high, threshold):
3. // If the size of the section is less than or equal to the threshold, use mergeSort
4. if (high - low + 1) <= threshold:
5. mergeSort(arr, low, high)
6. else:
7. // If the section is larger, proceed with quicksort
8. if low < high:
9. // Partition the array and get the pivot index
10. pivotIndex = partition(arr, low, high)
11. // Recursively apply hybridSort to the left part
12. hybridSort(arr, low, pivotIndex - 1, threshold)
13. // Recursively apply hybridSort to the right part
14. hybridSort(arr, pivotIndex + 1, high, threshold)
15. // Function to partition the array for quicksort
16. function partition(arr, low, high):
17. // Choose the pivot as the last element in the section
18. pivot = arr[high]
19. // Initialize the index of the smaller element
20. i = low - 1
21. // Loop through the section
22. for j = low to high - 1:
23. // If the current element is smaller than the pivot
24. if arr[j] < pivot:
25. // Move the index of the smaller element to the right
26. i = i + 1
27. // Swap the elements at indices i and j
28. swap(arr, i, j)
29. // Swap the pivot element to its correct position
30. swap(arr, i + 1, high)
31. // Return the index of the pivot element
32. return i + 1
33. // Function to implement merge sort
34. function mergeSort(arr, low, high):
35. // If there is more than one element in the section
36. if low < high:
37. // Find the middle index
38. mid = low + (high - low) / 2
39. // Recursively apply mergeSort to the left half
40. mergeSort(arr, low, mid)
41. // Recursively apply mergeSort to the right half
42. mergeSort(arr, mid + 1, high)
43. // Merge the sorted halves
44. merge(arr, low, mid, high)
45. // Function to merge two sorted halves
46. function merge(arr, low, mid, high):
47. // Calculate the size of the two halves
48. n1 = mid - low + 1
49. n2 = high - mid
50. // Create temporary arrays to hold the halves
51. left = createArray(n1)
52. right = createArray(n2)
53. // Copy the elements into the temporary arrays
54. for i = 0 to n1 - 1:
55. left[i] = arr[low + i]
56. for j = 0 to n2 - 1:
57. right[j] = arr[mid + 1 + j]
58. // Initialize indices for the temporary arrays and the main array
59. i = 0
60. j = 0
61. k = low
62. // Merge the temporary arrays back into the main array
63. while i < n1 and j < n2:
64. if left[i] <= right[j]:
65. arr[k] = left[i]
66. i = i + 1
67. else:
68. arr[k] = right[j]
69. j = j + 1
70. k = k + 1
71. // Copy any remaining elements from the left array
72. while i < n1:
73. arr[k] = left[i]
74. i = i + 1
75. k = k + 1
76. // Copy any remaining elements from the right array
77. while j < n2:
78. arr[k] = right[j]
79. j = j + 1
80. k = k + 1
81. // Function to swap two elements in the array
82. function swap(arr, i, j):
83. temp = arr[i]
84. arr[i] = arr[j]
85. arr[j] = temp
86. // Function to create an array of a given size
87. function createArray(size):
88. return new array of given size
89. // Main function to start the hybrid sorting process
90. function sort(arr):
91. // Determine the optimal threshold value for switching to mergeSort
92. threshold = determineOptimalThreshold()
93. // Call hybridSort on the entire array
94. hybridSort(arr, 0, arr.length - 1, threshold)
Detailed Explanation:
- Hybrid Sort Function:
- Line 2: This is the main function to implement the hybrid sorting algorithm.
- Line 3-4: Checks if the size of the current section of the array is less than or equal to the threshold. If true, it calls
mergeSort
on this section. - Line 6-8: If the section is larger than the threshold, it continues with the quicksort approach. It first checks if
low
is less thanhigh
to ensure there is more than one element to sort. - Line 9-10: Calls the
partition
function to partition the array and get the pivot index. - Line 11-12: Recursively calls
hybridSort
on the left part of the array. - Line 13-14: Recursively calls
hybridSort
on the right part of the array.
- Partition Function:
- Line 16: This function partitions the array for the quicksort algorithm.
- Line 18: Chooses the last element in the section as the pivot.
- Line 20: Initializes the index of the smaller element.
- Line 22-28: Loops through the section and swaps elements to ensure elements smaller than the pivot are on the left and larger elements are on the right.
- Line 30: Swaps the pivot element to its correct position.
- Line 32: Returns the index of the pivot element.
- Merge Sort Function:
- Line 34: This function implements the merge sort algorithm.
- Line 36: Checks if there is more than one element in the section.
- Line 38: Calculates the middle index of the section.
- Line 40: Recursively calls
mergeSort
on the left half of the array. - Line 42: Recursively calls
mergeSort
on the right half of the array. - Line 44: Merges the sorted halves.
- Merge Function:
- Line 46: This function merges two sorted halves of the array.
- Line 48: Calculates the size of the two halves.
- Line 50: Creates temporary arrays to hold the elements of the two halves.
- Line 54-57: Copies the elements into the temporary arrays.
- Line 59-61: Initializes indices for the temporary arrays and the main array.
- Line 63-70: Merges the elements back into the main array by comparing elements from the temporary arrays and placing the smaller one first.
- Line 72-75: Copies any remaining elements from the left temporary array.
- Line 77-80: Copies any remaining elements from the right temporary array.
- Swap Function:
- Line 82: This function swaps two elements in the array.
- Line 83-85: Swaps the elements at indices
i
andj
.
- Create Array Function:
- Line 87: This function creates an array of a given size.
- Line 88: Returns a new array of the specified size.
- Main Sort Function:
- Line 90: This is the main function to start the hybrid sorting process.
- Line 92: Determines the optimal threshold value for switching to merge sort.
- Line 94: Calls
hybridSort
on the entire array starting from index 0 to the last index.
Conclusion
The hybrid sorting algorithm that combines Quicksort and Mergesort represents a significant advancement in the realm of sorting techniques. By leveraging the rapid partitioning and in-place sorting capabilities of Quicksort for larger datasets and the stable, consistent performance of Mergesort for smaller partitions, this hybrid approach addresses the limitations inherent in both algorithms. The result is a robust and efficient sorting solution that can adapt to various dataset sizes and structures, providing optimal performance across a wide range of scenarios.
Throughout this article, we have explored the underlying principles and mechanics of Quicksort and Mergesort, setting the stage for a deeper understanding of the hybrid algorithm. The detailed pseudocode and step-by-step explanations have illustrated how these two sorting methods can be seamlessly integrated, ensuring clarity and ease of implementation. By carefully managing the threshold for switching between Quicksort and Mergesort, we can fine-tune the hybrid algorithm to achieve the best possible performance, balancing speed and stability.
The hybrid sorting algorithm not only demonstrates the power of combining multiple techniques but also underscores the importance of adaptability in algorithm design. In a world where data continues to grow in volume and complexity, the ability to efficiently sort and organize information is more critical than ever. This hybrid approach offers a practical and effective solution, equipping developers and computer scientists with the tools they need to handle diverse and demanding sorting tasks.
Moreover, the insights gained from implementing and understanding this hybrid algorithm extend beyond sorting. They provide valuable lessons in algorithm optimization, recursion management, and the trade-offs between time and space complexity. These principles are applicable to a wide range of computational problems, highlighting the broader significance of the hybrid sorting algorithm.
In conclusion, the hybrid sorting algorithm combining Quicksort and Mergesort exemplifies the innovative spirit of computer science, where the blending of different techniques can lead to powerful and efficient solutions. By embracing the strengths of both Quicksort and Mergesort, this hybrid approach not only enhances sorting performance but also inspires new ways of thinking about algorithm design and optimization. As data continues to evolve, so too must our approaches to managing it, and the hybrid sorting algorithm stands as a testament to the ongoing pursuit of excellence in the field of computer science.