Introduction
In the ever-evolving landscape of technology, full stack development has emerged as one of the most dynamic and comprehensive disciplines in the software development field. The term “full stack developer” signifies an individual who possesses a broad skill set that spans both the front-end and back-end of web development, encompassing everything from user interface design to server-side logic and database management. As businesses and organizations increasingly rely on sophisticated web applications to deliver seamless user experiences and drive innovation, the demand for proficient full stack developers continues to soar.
Mastering full stack development is no small feat; it requires a deep understanding of a myriad of technologies and methodologies that work in harmony to create robust and scalable web applications. This journey involves navigating through various layers of the tech stack, including HTML, CSS, and JavaScript for front-end development, and server-side languages, frameworks, and databases for back-end development. Furthermore, aspiring full stack developers must also gain expertise in crucial areas such as API development, deployment, and DevOps practices. The breadth of knowledge required is vast, encompassing not only technical skills but also soft skills such as problem-solving, communication, and continuous learning.
The roadmap to becoming a full stack developer is both challenging and rewarding. It involves a structured approach to learning and mastering a wide range of tools and technologies, each of which plays a pivotal role in the development lifecycle. From understanding core technologies like HTML and CSS, and mastering JavaScript frameworks, to exploring server-side languages and database management, every step in this journey is integral to building a comprehensive skill set. Additionally, proficiency in deployment, monitoring, and professional development ensures that full stack developers are equipped to handle real-world scenarios and deliver high-quality applications.
This article aims to provide a detailed and structured pseudo code guide for aspiring full stack developers. By offering a diversified roadmap that includes multiple options at each stage of the learning process, the guide ensures that developers can tailor their learning path to their preferences and career goals. Whether you are just starting out in the field or looking to deepen your expertise, this guide will help you navigate the complexities of full stack development and achieve mastery in this multifaceted discipline.
Enhanced Pseudo Code for Becoming a Full Stack Developer
This enhanced pseudo code provides a diversified roadmap for mastering full stack development. It offers multiple options at each stage to accommodate different learning preferences and technology choices. By exploring a range of tools, languages, and frameworks, aspiring developers can tailor their learning path to their interests and career goals.
1. Understand Core Technologies
1.1 Learn HTML/CSS
function LearnHTMLCSS():
// Learn basic HTML
Read("HTML Basics Tutorial")
Practice("Create a simple web page with headings, paragraphs, and lists")
// Learn advanced HTML
Read("HTML5 Features and Elements")
Practice("Use forms, multimedia elements, and semantic tags")
// Learn basic CSS
Read("CSS Fundamentals")
Practice("Style the web page with colors, fonts, and layouts")
// Learn advanced CSS
Read("CSS Flexbox and Grid")
Practice("Create responsive layouts with Flexbox and Grid")
// Optional: Learn CSS preprocessors
Choose("Sass or LESS")
Read("CSS Preprocessor Documentation")
Practice("Use Sass or LESS to enhance CSS workflows")
1.2 Master JavaScript
function LearnJavaScript():
// Basics of JavaScript
Read("JavaScript Basics")
Practice("Write basic scripts to manipulate the DOM")
// Advanced JavaScript
Read("Asynchronous JavaScript and Promises")
Practice("Handle asynchronous operations with promises and async/await")
// Modern JavaScript Features
Read("ES6+ Features")
Practice("Use arrow functions, destructuring, and template literals")
// JavaScript Frameworks
Choose("React or Angular or Vue or Svelte")
Read("Framework Documentation")
Practice("Build a small project using the chosen framework")
// Optional: Learn TypeScript
Learn("TypeScript Basics")
Practice("Use TypeScript with JavaScript frameworks")
2. Front-End Development
2.1 Learn a Front-End Framework
function LearnFrontEndFramework(framework):
if framework == "React":
Read("React Documentation")
Practice("Build components and manage state with hooks")
Learn("React Native for mobile development")
else if framework == "Angular":
Read("Angular Documentation")
Practice("Create components and use Angular services")
Learn("Angular CLI and Angular Material")
else if framework == "Vue":
Read("Vue Documentation")
Practice("Build components and manage state with Vuex")
Learn("Vue Router for routing")
else if framework == "Svelte":
Read("Svelte Documentation")
Practice("Build reactive components and use SvelteKit for full-stack development")
2.2 Responsive Design and UX
function LearnResponsiveDesign():
Read("Responsive Web Design Principles")
Practice("Create responsive layouts using media queries and flexible grids")
// Optional: Learn Mobile-First Design
Read("Mobile-First Design Strategy")
Practice("Implement mobile-first approaches in your designs")
function LearnUXDesign():
Read("User Experience Design Basics")
Practice("Create wireframes and prototypes using tools like Figma or Adobe XD")
Conduct("Usability Testing and Gather Feedback")
// Optional: Learn about Accessibility (a11y)
Read("Web Accessibility Standards")
Practice("Implement accessibility features and test your designs")
3. Back-End Development
3.1 Choose a Server-Side Language
function LearnServerSideLanguage(language):
if language == "Node.js":
Read("Node.js Basics and Express Framework")
Practice("Build RESTful APIs and manage routes")
Learn("Express Middleware and Error Handling")
else if language == "Python":
Read("Python Basics and Flask or Django")
Practice("Create web applications and work with ORM")
Learn("Flask Extensions or Django REST Framework")
else if language == "Ruby":
Read("Ruby Basics and Rails Framework")
Practice("Build CRUD applications and use ActiveRecord")
Learn("Rails API Mode and Gem Ecosystem")
else if language == "Java":
Read("Java Basics and Spring Boot")
Practice("Create microservices and handle dependency injection")
Learn("Spring Security and Spring Data")
3.2 Database Management
function LearnDatabaseManagement(dbType):
if dbType == "Relational":
Read("SQL Basics and Advanced Queries")
Practice("Design schema and perform CRUD operations with PostgreSQL or MySQL")
Learn("Database Indexing and Optimization Techniques")
else if dbType == "NoSQL":
Read("NoSQL Basics and Document Databases")
Practice("Design schema and perform CRUD operations with MongoDB or Cassandra")
Learn("Data Sharding and Replication Techniques")
4. API Development and Integration
4.1 Learn RESTful API Development
function LearnRESTfulAPIs():
Read("REST Architecture Principles")
Practice("Design and implement RESTful endpoints")
Test("APIs using tools like Postman or Swagger")
// Optional: Learn API Versioning
Read("API Versioning Strategies")
Practice("Implement API versioning in your RESTful services")
4.2 Explore GraphQL
function LearnGraphQL():
Read("GraphQL Basics and Schema Definition")
Practice("Create a GraphQL schema and resolvers")
Test("GraphQL queries and mutations")
// Optional: Learn GraphQL Subscriptions
Read("GraphQL Subscriptions for Real-Time Data")
Practice("Implement real-time features using subscriptions")
5. Deployment and DevOps
5.1 Version Control
function LearnVersionControl():
Read("Git Basics and Workflow")
Practice("Use Git for version control and collaborate on GitHub or GitLab")
Learn("Branching Strategies and Merging Techniques")
5.2 Containerization
function LearnContainerization():
Read("Docker Basics and Dockerfiles")
Practice("Create and manage Docker containers")
Learn("Kubernetes for orchestration and deployment")
// Optional: Learn Docker Compose for multi-container setups
Learn("Docker Compose Basics")
Practice("Manage multi-container applications with Docker Compose")
5.3 CI/CD Pipelines
function LearnCICDPipelines():
Read("CI/CD Concepts and Tools")
Practice("Set up pipelines using Jenkins, GitHub Actions, or GitLab CI")
Learn("Pipeline as Code and Deployment Strategies")
// Optional: Learn about Infrastructure as Code (IaC)
Read("Infrastructure as Code Basics with Terraform or AWS CloudFormation")
Practice("Automate infrastructure provisioning with IaC tools")
5.4 Monitoring and Performance
function LearnMonitoringAndPerformance():
Read("Application Monitoring Tools")
Practice("Implement monitoring with Prometheus or New Relic")
Optimize("Application performance through caching and profiling")
// Optional: Learn about Distributed Tracing
Read("Distributed Tracing with tools like Jaeger or Zipkin")
Practice("Implement tracing for complex microservices architectures")
6. Soft Skills and Professional Development
6.1 Problem-Solving Skills
function DevelopProblemSolvingSkills():
Practice("Solve coding challenges and participate in hackathons")
Read("Problem-Solving Techniques and Strategies")
// Optional: Learn Algorithms and Data Structures
Read("Algorithms and Data Structures Basics")
Practice("Implement and solve problems using common algorithms and data structures")
6.2 Communication Skills
function ImproveCommunicationSkills():
Practice("Explain technical concepts clearly and concisely")
Participate("In team meetings and code reviews")
// Optional: Learn about Technical Writing
Read("Technical Writing Basics")
Practice("Document code and write technical documentation")
6.3 Continuous Learning
function EngageInContinuousLearning():
Read("Latest Industry Trends and Technologies")
Take("Online courses, attend conferences, and join tech communities")
// Optional: Join Professional Networks and Associations
Join("Professional Developer Networks and Associations")
Network("Connect with peers and mentors in the industry")
6.4 Team Collaboration
function EnhanceTeamCollaboration():
Practice("Work in collaborative projects and contribute to open source")
Develop("Skills for effective teamwork and conflict resolution")
// Optional: Learn Agile and Scrum Methodologies
Read("Agile and Scrum Basics")
Practice("Participate in Agile/Scrum ceremonies and practices")
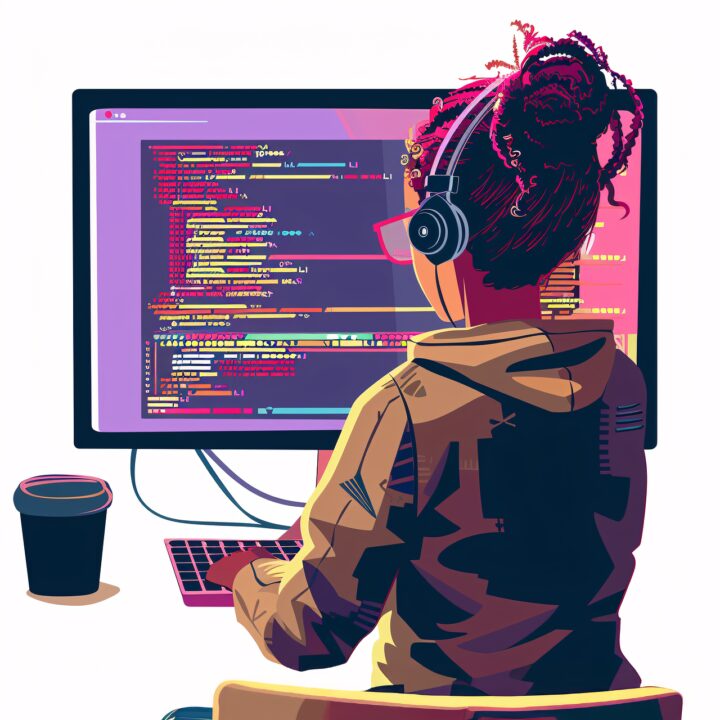
Explanation of the Pseudo Code
1. Understand Core Technologies
- Learn HTML/CSS: Start by mastering the fundamental building blocks of web development. HTML and CSS are crucial for creating and styling web pages. Basic HTML and CSS are necessary for any web development, while advanced CSS techniques like Flexbox and Grid enhance the design’s responsiveness. Learning CSS preprocessors like Sass or LESS provides additional tools for more efficient CSS management.
- Master JavaScript: JavaScript is essential for making web pages interactive. Begin with basic JavaScript and advance to more complex topics like asynchronous programming and modern JavaScript features (ES6+). Choose a framework or library to specialize in, such as React, Angular, Vue, or Svelte, and consider learning TypeScript for enhanced JavaScript development.
2. Front-End Development
- Learn a Front-End Framework: Specializing in a front-end framework allows you to build more dynamic and scalable user interfaces. Each framework has its unique features and strengths—React for component-based architecture, Angular for a comprehensive framework, Vue for simplicity and flexibility, and Svelte for a reactive approach. Additionally, exploring mobile development or other framework features can broaden your expertise.
- **Responsive Design and UX**: Mastering responsive design ensures that web applications work well on various devices. Learning UX design principles helps create user-friendly and aesthetically pleasing interfaces. Understanding accessibility ensures your applications are usable by people with disabilities. Optional skills like mobile-first design and UX research enhance your design capabilities.
3. Back-End Development
- Choose a Server-Side Language: The back end of a web application handles server-side logic and data management. Each language offers different frameworks and libraries: Node.js with Express, Python with Flask or Django, Ruby with Rails, and Java with Spring Boot. Choose a language that aligns with your career goals and interests, and learn additional tools and libraries to enhance your backend development skills.
- Database Management: Understanding both relational and NoSQL databases is crucial for data management. Relational databases like PostgreSQL and MySQL require knowledge of SQL and schema design, while NoSQL databases like MongoDB offer a more flexible data model. Learning about database optimization, sharding, and replication techniques can improve performance and scalability.
4. API Development and Integration
- Learn RESTful API Development: APIs allow different software systems to communicate. Learning RESTful principles and practices helps you create robust APIs. Tools like Postman or Swagger assist in testing and documenting APIs. Understanding API versioning and integrating GraphQL for more flexible data querying can enhance your API development skills.
- Explore GraphQL: GraphQL is an alternative to RESTful APIs that offers more flexibility in querying and mutating data. Learning GraphQL involves understanding its schema definition and resolver implementation, as well as exploring advanced features like subscriptions for real-time data.
5. Deployment and DevOps
- Version Control: Git is essential for managing code changes and collaborating with others. Understanding branching strategies and version control workflows is crucial for effective team collaboration and code management.
- Containerization: Docker and container orchestration with Kubernetes are key for modern application deployment. Docker simplifies application deployment by packaging code and dependencies into containers. Kubernetes manages and scales containerized applications. Learning Docker Compose can simplify multi-container setups.
- CI/CD Pipelines: Continuous Integration and Continuous Deployment (CI/CD) automate the process of testing and deploying code changes. Tools like Jenkins, GitHub Actions, and GitLab CI help set up pipelines. Knowledge of Infrastructure as Code (IaC) with tools like Terraform or CloudFormation can further automate infrastructure management.
- Monitoring and Performance: Monitoring tools like Prometheus or New Relic track application performance and health. Optimizing performance through caching and profiling, and understanding distributed tracing, can help maintain high-performance applications.
6. Soft Skills and Professional Development
- Problem-Solving Skills: Strong problem-solving skills are essential for tackling coding challenges and developing efficient solutions. Participating in hackathons and learning algorithms and data structures can enhance these skills.
- Communication Skills: Effective communication is vital for explaining technical concepts and collaborating with team members. Technical writing skills help document code and create clear documentation.
- Continuous Learning: Staying updated with industry trends and technologies through courses, conferences, and professional networks is crucial for ongoing development. Joining professional associations and networking with peers can provide additional career growth opportunities.
- Team Collaboration: Working effectively in teams and contributing to open-source projects enhances collaboration skills. Learning Agile and Scrum methodologies can further improve teamwork and project management.
This enhanced pseudo code provides a comprehensive framework for becoming a full stack developer, incorporating a wide range of technologies, tools, and skills. By following this roadmap, developers can build a robust and versatile skill set, paving the way for a successful career in full stack development.
Conclusion
The journey to becoming a full stack developer is one marked by continuous learning, adaptability, and a commitment to mastering a broad spectrum of technologies. As web development continues to advance and evolve, the role of the full stack developer remains central to creating innovative and effective web applications. The comprehensive pseudo code provided in this article serves as a detailed roadmap for navigating this intricate path, offering a structured approach to learning and mastering the diverse skills required for success.
By following the roadmap, aspiring developers can gain proficiency in essential technologies such as HTML, CSS, and JavaScript, while also exploring a variety of frameworks, libraries, and tools. The guide emphasizes the importance of understanding both front-end and back-end development, as well as the integration of APIs, deployment strategies, and DevOps practices. Additionally, the inclusion of optional learning paths and advanced topics ensures that developers can tailor their learning journey to their individual interests and career aspirations.
Ultimately, the role of a full stack developer is not just about technical proficiency; it also involves cultivating problem-solving skills, effective communication, and a dedication to ongoing professional development. As the tech industry continues to evolve, full stack developers must stay abreast of the latest trends and technologies to remain competitive and deliver exceptional results.
In conclusion, the path to becoming a full stack developer is both demanding and rewarding. It requires a commitment to mastering a diverse array of skills and technologies, along with a willingness to continuously adapt and learn. By following the detailed pseudo code roadmap outlined in this article, developers can build a solid foundation and advance their careers in this exciting and ever-changing field. With dedication, practice, and a passion for innovation, aspiring full stack developers can achieve success and make significant contributions to the world of web development.